Introduction to OpenGL
Tom Kelliher, CS 320
Feb. 16, 2000
Monday is ``lab day.''
Have a look at, and run, viewport.c and paint.c (available on the class
home page).
Finished primitive basics.
- Color and color models.
- 2-D viewing.
- OpenGL control functions.
Viewports, interaction.
Additive color model:

Tristimulus value.
Notes:
- Color is continuous.
- Our visual system perceives light as a three-color system.
- Basic tenet of three-color theory: If two colors produce the
same tristimulus values, then they are visually indistinguishable.
- Additive: RGB. Subtractive: CMY.
- OpenGL has two color modes: RGB and indexed.
- Depth of the frame buffer. True color: 24 bits. Number of
colors?
- We specify a color as a point within the color cube:
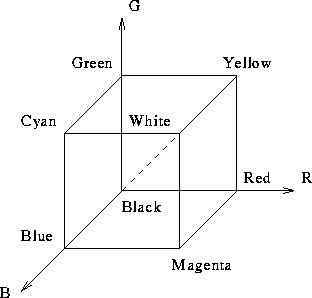
glColor3f(1.0, 0.0, 0.0);
- Alpha value provides opacity factor:
glClearColor(1.0, 1.0, 1.0, 1.0);
- Many frame buffers have limited depth, say eight bits. However, the
graphics controller may be able to display 16M colors.
- Suppose: frame buffer has depth k bits. Each color is specified
using m bits.
At any point in time, we should be able to specify any
colors from
the total collection of
colors.
Use of a color-lookup table:
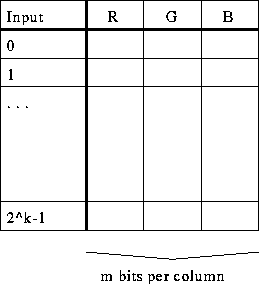
- Shading, for 3-D graphics, requires a large # of colors. So, we'll
stick with RGB mode.
2-D viewing:
- Objects inside the viewing/clipping rectangle are visible:
What is clipping?
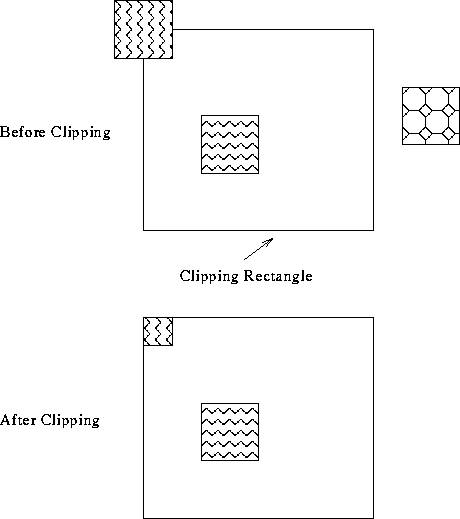
- The default viewing volume is
and centered at
the origin.
The orthographic projection:
- Projects the point
onto
.
- In 2-D, we generally want to place objects at z=0.
- OpenGL specification:
void glOrtho(GLdouble left, GLdouble right,
GLdouble bottom, GLdouble top,
GLdouble near, GLdouble far);
void glOrtho2d(GLdouble left, GLdouble right,
GLdouble bottom, GLdouble top);
``Multiply the current matrix with an orthographic matrix'' --- make the
projection matrix the current matrix.
- The OpenGL camera defaults to being positioned at the origin, pointed
in the -z direction. The camera can ``see'' behind itself.
OpenGL matrices:
- Modelview matrix: positions objects in front of the camera.
Translation, rotation, scaling.
- Projection matrix: determines shape of the viewing volume.
Orthographic, perspective.
Several we've already seen:
-
glutInit(int *argcp, char *argv[])
-
glutCreateWindow(char *title)
-
glutInitDisplayMode(unsigned int mode)
Example:
glutInitDisplayMode(GLUT_RGB | GLUT_DEPTH | GLUT_DOUBLE);
Mode bit examples:
-
GLUT_RGBA
, GLUT_RGB
, GLUT_INDEX
.
-
GLUT_SINGLE
, GLUT_DOUBLE
.
-
GLUT_DEPTH
.
Thomas P. Kelliher
Wed Feb 16 09:35:24 EST 2000
Tom Kelliher