Introduction to OpenGL
Tom Kelliher, CS 320
Feb. 11, 2000
Read 2.5--2.6.
Project due 2/25.
Vertex specification, coordinate systems.
-
Viewing and Control.
Support provided by a graphics API:
- Primitive functions: points, line segments, polygons, text, curves,
surfaces.
- Attribute functions: color, fill, type face.
- Viewing functions: attributes of the synthetic camera.
- Transformation functions: rotation, translation, scaling. Matrix
computations.
- Input functions: keyboard, pointing device.
- System communication: window system, OS, other workstations, other
users.
OpenGL Library Organization:
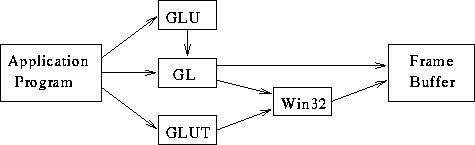
Consider:
glBegin(primitiveType)
glVertex*( ... );
// ...
glVertex*( ... );
glEnd();
for the primitiveTypes:
-
GL_POINTS
.
-
GL_LINES
.
-
GL_LINE_STRIP
.
-
GL_LINE_LOOP
. When is this not a polygon?
- Polygons have interiors which can be filled.
- What is filling? Color, pattern (bitmap) fills.
- Simple polygon: no edges cross.
- When filling, how do we determine if a point is interior? Crossing
test.
- Fill example: five-pointed star. Scanlines. Is this the effect we
want?
- Winding test improvement:
- Pick any vertex and direction.
- Traverse the polygon, labeling each edge with direction of
traversal.
- Scanline crosses ``up'' edge: add 1. Crosses ``down'' edge:
subtract 1.
- Point's winding number starts at 0. In interior if final winding
number not 0.
- Complications: lines parallel to scanline, intersections with
vertices.
- Convex and concave polygons.
- Definitions.
- Concave polygons can present problems.
- Often, hardware and software supports triangles.
- 2-D polygon convexity test: for any interior point, it is ``to
the right'' when traversing the edges clockwise.
- OpenGL polygon types:
GL_POLYGON
, GL_TRIANGLES
,
GL_QUADS
, GL_TRIANGLE_STRIP
, GL_QUAD_STRIP
,
GL_TRIANGLE_FAN
.
What are the strip and fan types?
- Stroke text:
- Specified by vertices.
- Can send it through the graphics pipeline to manipulate it (point
size, italics face).
- Computational-, storage-expensive
- Example: Postscript.
- Raster text:
- Specified by a bit-map.
- A font is often stored as a strip. Individual characters are
copied to the frame buffer via bitblt operations.
- Can't process through the graphics pipeline. Changing the point
size by changing the pixel size: blockiness. Or by using several
``strips.''
- Computational-, storage-inexpensive.
Tessellation: Mesh of convex polygons. 2-D, 3-D.
Example: circle formed by GL_TRIANGLE_FAN
.
Additive color model:

Tristimulus value.
Notes:
- Color is continuous.
- Our visual system perceives light as a three-color system.
- Basic tenet of three-color theory: If two colors produce the
same tristimulus values, then they are visually indistinguishable.
- Additive: RGB. Subtractive: CMY.
- OpenGL has two color modes: RGB and indexed.
- Depth of the frame buffer. True color: 24 bits. Number of
colors?
- We specify a color as a point within the color cube:
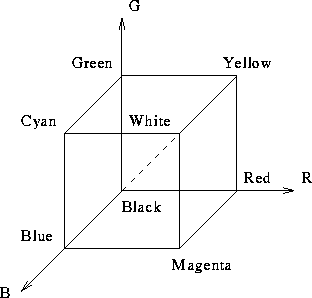
glColor3f(1.0, 0.0, 0.0);
- Alpha value provides opacity factor:
glClearColor(1.0, 1.0, 1.0, 1.0);
- Many frame buffers have limited depth, say eight bits. However, the
graphics controller may be able to display 16M colors.
- Suppose: frame buffer has depth k bits. Each color is specified
using m bits.
At any point in time, we should be able to specify any
colors from
the total collection of
colors.
Use of a color-lookup table:
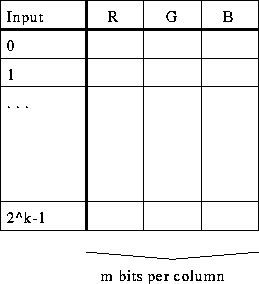
- Shading, for 3-D graphics, requires a large # of colors. So, we'll
stick with RGB mode.
Thomas P. Kelliher
Thu Feb 10 21:43:14 EST 2000
Tom Kelliher