Virtual Memory I
Tom Kelliher, CS42
Nov. 1, 1996
- Virtual memory --- what is it?
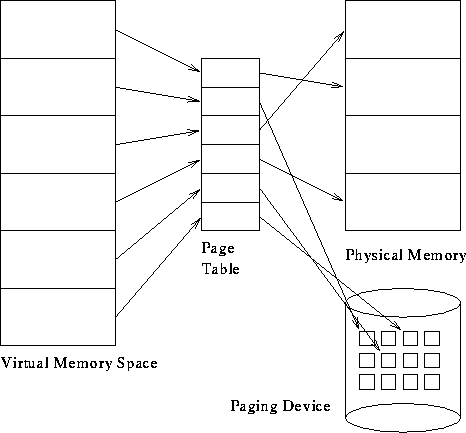
- What are the advantages?
- A program's logical address space can be larger than physical
memory.
- Degree of multiprogramming can be increased (40 pages of memory;
allocate only 5 pages to processes with spaces of 10 pages).
- Less I/O needed to load/swap a process.
- Demand paging.
- Why does it work?
- A lot of code is rarely run (error-handling routines).
- Oversizing of data structures.
- Locality of reference:
- Spatial.
- Temporal.
What we'll consider:
- System support.
- Page fault sequence.
- Replacement policies.
- Placement (allocation) policies.
- Kernel support.
- MMU support.
- CPU support.
- Page fault handler.
- Page placement policies.
- Page replacement policies.
Page table changes:
- Valid/invalid bit takes on greater role:
- Valid: frame field contains frame number.
- Invalid: frame field contains block number on paging device
or invalid reference.
- Dirty bit: page has been modified.
- Read/write bit.
- Access counter: (sometimes) for implementing LRU.
Traps generated:
- Memory fault.
- Page fault.
- Write on read-only fault.
Instructions must be restartable:
- May page fault on instruction fetch.
- May page fault on operand fetch/store. State may have been modified.
Design approaches:
- Checkpoint state.
- Ensure that all required pages are in memory before proceeding.
- Memory reference generated.
- MMU looks up Page table entry (TLB then memory).
- Valid bit examined. If set, get frame number and finish.
- Otherwise, generate page fault trap.
- Kernel page fault handler called as result of trap.
- Kernel examines page table entry.
- If non-mapped, generate page violation trap.
- Otherwise, locate a frame for the incoming page (possibly designate a
victim frame and page it out first).
- Schedule disk I/O.
- Re-schedule CPU.
- Disk I/O completes, interrupt generated.
- Kernel interrupt handler called and determines source of interrupt.
- Update page table and put process back on ready queue.
- Faulting instruction re-started.

where:
- p is the page fault rate. 25 ms or more.
- ma is main memory access time. 100 ns or less.
- If page fault rate is 1/1,000, effective access time is
25 microseconds!!!
- If we want only a 10% penalty (110 ns), page fault rate must be less
than 1/2,500,000.
- Copy image into swap at process start-up. Demand paging done from
swap device. Wastes swap space, extra I/O, but swap device is faster than
filesystem.
- Demand page from filesystem. Read-only pages are never swapped out,
just overwritten and re-read from filesystem. Conserves swap space, uses
slower filesystem.
- Demand page from filesystem, swap out to swap device. Only demanded
pages are read from filesystem, only necessary pages are replaced to swap
device.
- What happens if a page fault occurs and all frames are in use?
- Must select a victim frame:
- Page-out victim frame.
- Update victim process' page table.
- Page-in faulted page.
- How do we select the victim frame?
- Comparison criteria for replacement algorithms.
- What is it?
- Where do I get one?
- What about redundancy?
- In the set of candidate victim pages, select the ``oldest'' page.
- Example reference string: 7, 0, 1, 2, 0, 3, 0, 4, 2, 3, 0, 3, 2, 1,
2, 0, 1, 7, 0, 1. Three frames allocated.
- Belady's anomaly:
- Reference string: 1, 2, 3, 4, 1, 2, 5, 1, 2, 3, 4, 5.
- Three frames allocated.
- Four frames allocated.
- Stack property: Set of pages in memory with n frames allocated is a
subset of set of pages in memory with n + 1 frames allocated.
- Replace the page which won't be used for the longest time.
- Example reference string: 7, 0, 1, 2, 0, 3, 0, 4, 2, 3, 0, 3, 2, 1,
2, 0, 1, 7, 0, 1. Three frames allocated.
- Implementation?
- Approximation to optimal: replace page which hasn't been used for the
longest time.
- ``Reversal'' of optimal.
- Example reference string: 7, 0, 1, 2, 0, 3, 0, 4, 2, 3, 0, 3, 2, 1,
2, 0, 1, 7, 0, 1. Three frames allocated.
- Implementation:
- Counters. Hardware support required
- Stack. Expensive.
- Reference bits concatenation as approximation to counter.
- Second chance (clock) algorithm: FIFO, but skip over page if
reference bit set (reset reference bit).
- Keep a small pool of empty frames so paging-in can occur without
waiting for victim page-out.
- When idle, write dirty pages out and clear dirty bit.
- Keep track of what's in free frames, so page-ins can possibly use an
old, free frame.
Thomas P. Kelliher
Thu Oct 31 22:50:32 EST 1996
Tom Kelliher