Introduction to OpenGL
Tom Kelliher, CS 320
Feb. 2, 1998
Announcements:
From last time:
- Programmer's interface.
- Pictorial history of computer graphics.
- Modeling-Rendering paradigm.
- Graphics architectures.
Outline:
- Introduction: Sierpinski gasket, pen-plotter model, vertex
specification, coordinate systems.
- OpenGL API.
- Primitive basics: points, line segments, polygons.
Assignment: Browse the on-line resources and the man pages on phoenix. In
particular, look at the
OpenGL Programming Guide
.
Consider the Sierpinski gasket problem for a triangle:
// Initialize.
clear the viewing window;
set vertices of the triangle;
let currentPoint = random point in interior of triangle;
randomly select one of the three triangle vertices;
// Run.
while (notDone)
{
nextPoint = point half-way between currentPoint and
selected vertex;
display nextPoint;
currentPoint = nextPoint;
randomly select one of the three triangle vertices;
}
Questions:
- In what coordinate system do we work?
- In how many dimensions?
Note that all our output devices are 2-D.
- Uses a gantry to move a pen.
- Gantry moves in x and y.
- Pen can be raised and lowered.
- Operations:
-
moveto(x, y);
-
lineto(x, y);
- This model is still in use with pen-plotters and PDLs such as
Postscript.
- How do we extend to 3-D?
- Must compute the 2-D projection of 3-D points. (Application of
trigonometry, which you should review for later.)
- Should application or API perform the projections?
- We can set z=0 so that we're working 2-D in 3-D.
Can't do much without vertices.
Indicator for the general form: glVertex*()
(see man page on
phoenix for glvertex
.
Some examples:
-
glVertex2i(GLint xi, GLint yi);
-
glVertex3f(GLfloat x, GLfloat y);
- Using an array to store the vertex coordinates:
GLfloat vertex[3] = { 0.0f, 1.0f, 2.0f };
glVertex3fv(vertex);
Vertices are grouped using glBegin()
/glEnd()
:
glBegin(GL_LINES);
glVertex2f(x1, y1);
glVertex2f(x2, y2);
glVertex2f(x3, y3);
glVertex2f(x4, y4);
glEnd();
or
glBegin(GL_POINTS);
glVertex2f(x1, y1);
glVertex2f(x2, y2);
glVertex2f(x3, y3);
glVertex2f(x4, y4);
glEnd();
Heart of the Sierpinski gasket program:
void display(void)
{
static point2 p = { x, y }; /* Initial point.
int i;
i = rand() % 3;
p[0] = (p[0] + triangle[0]) / 2.0;
p[1] = (p[1] + triangle[1]) / 2.0;
glBegin(GL_POINTS);
glVertex2fv(p);
glEnd();
}
What are our units for x, y, and z?
- Originally, graphics systems worked in coordinates of display device.
- Portability?
- Much more convenient to work in problem coordinates, let API convert:
device independence.
- Mapping from world coordinates to device coordinates. For us, window
coordinates.
Support provided by a graphics API:
- Primitive functions: points, line segments, polygons, text, curves,
surfaces.
- Attribute functions: color, fill, type face.
- Viewing functions: attributes of the synthetic camera.
- Transformation functions: rotation, translation, scaling. Matrix
computations.
- Input functions: keyboard, pointing device.
- System communication: window system, OS, other workstations, other
users.
OpenGL Library Organization:
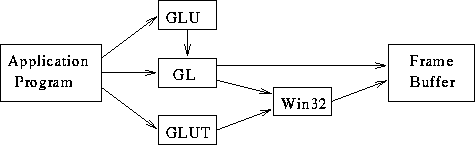
Consider:
glBegin(primitiveType)
glVertex*( ... );
// ...
glVertex*( ... );
glEnd();
for the primitiveTypes:
-
GL_POINTS
.
-
GL_LINES
.
-
GL_LINE_STRIP
.
-
GL_LINE_LOOP
. When is this not a polygon?
- Polygons have interiors which can be filled.
- What is filling? Color, pattern (bitmap) fills.
- Simple polygon: no edges cross.
- When filling, how do we determine if a point is interior? Crossing
test.
- Fill example: five-pointed star. Scanlines. Is this the effect we
want?
- Winding test improvement:
- Pick any vertex and direction.
- Traverse the polygon, labeling each edge with direction of
traversal.
- Scanline crosses ``up'' edge: add 1. Crosses ``down'' edge:
subtract 1.
- Point's winding number starts at 0. In interior if final winding
number not 0.
- Complications: lines parallel to scanline, intersections with
vertices.
- Convex and concave polygons.
- Definitions.
- Concave polygons can present problems.
- Often, hardware and software supports triangles.
- 2-D polygon convexity test: for any interior point, it is ``to
the right'' when traversing the edges clockwise.
Thomas P. Kelliher
Thu Jan 29 13:04:13 EST 1998
Tom Kelliher