Architectural Support for Modern Operating Systems
Tom Kelliher, CS 318
Jan. 23, 1998
From last time:
- OS as interface.
- Layering and abstractions.
- Historical developments. Why multiprogram a single user
workstation?
Outline:
-
Read Chapter 3.
Consider the contrived organization:
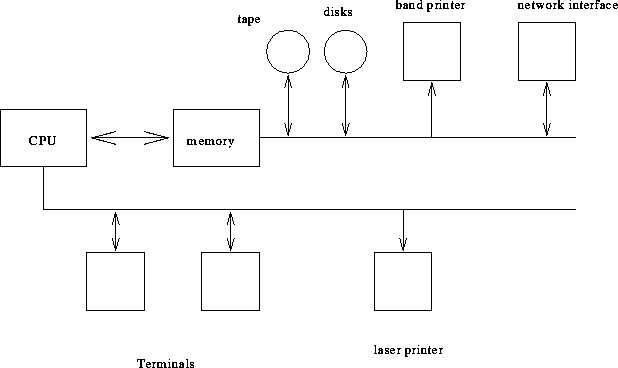
How does the CPU manage all these resources?
What are their relative speeds?
- CPU: 300,000,000 instructions/sec.
- Memory: 10,000,000 word accesses/sec.
- Terminal: 4 characters/sec.
- Disk: 10Mb/sec; 10 ms latency
- Typical network card: 1Mb/sec.
- ``Future'' network cards: 100Mb/sec., 1Gb/sec.
A small example:
- Ethernet data arriving at 1Gb/sec. with an MTU of 1500. 7E7
packets/sec.
- 100 instructions in packet interrupt routine.
- 300MHz processor.
- 23% of processor cycles needed to handle packets.
How is data transferred between outside world and memory?
CPU constantly checks on status of pending I/O device requests.
Consider a kernel level routine for reading a disk block:
int ReadBlock (disk d, char* buffer, int blockNum)
{
while (d.busy) /* Verify idle */
/* Go do something else and check back. */
/* Send read command to disk. */
while (d.busy)
/* Go do something else and check back. */
return d.status;
}
How safe is this? How efficient is it?
- The I/O device itself signals CPU when an operation completes.
- Less burden for CPU.
- Cpu must determine:
- Source of interrupt.
- Interrupt type.
- (Possibly) Transfer data.
- Byte devices and block devices.
Introduce parallelism into system by decoupling CPU
and I/O devices. (Asynchronous vs. synchronous.)
Schema:
- Start device.
- Go do something useful.
- Device signals completion via interrupt.
- Kernel handles interrupt.
Interrupt Features:
- Current process is temporarily abandoned --- arbitrary function call.
- Several priority levels.
- Most levels can be masked.
- Several devices can share the same interrupt line. How do we
identify the sender?
- Kernel contains interrupt handlers which service interrupts.
How do they identify the device if several exist?
- After handler finished, unmask interrupts and return to previous
process.
(Direct Memory Access.)
A block I/O device could swamp a CPU forced to perform the actual
data transfers.
Solution: Let device access memory and transfer data itself.
Here's what's going on with DMA:
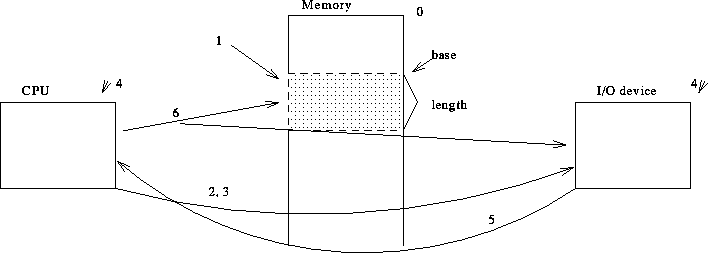
- CPU reserves an area of memory as a buffer for the I/O (assume a read
is performed).
- CPU loads the base (bottom) address of the buffer into the I/O device.
- CPU loads the length of the transfer into the I/O device (assume that
it's same as the buffer size).
- Concurrently:
- CPU goes on to another task (block requesting process).
- I/O device starts transfer, writes data directly to memory.
Memory arbitration problems here.
- I/O device interrupts CPU upon completion.
- CPU receives interrupt, checks status, schedules formerly blocked
process.
Storage hierarchy:
- Registers.
- Caches.
- Main Memory.
- Disk (secondary storage).
- Flavors: electronic, magnetic, optical, removable, etc.
- Tape (tertiary storage).
Points to consider: size, speed, cost, degree of OS management.
- Dual mode operation.
- Privileged instructions.
- Memory protection mechanisms.
- Interval timers.
CPU operates in two states:
- Supervisor mode
- All instructions may be executed.
- This is a privileged mode.
- Only parts of the OS run in this mode.
- User threads never run directly in this mode.
- User mode
- Some instructions are ``privileged.''
- Attempt to execute a privileged instruction results in a trap.
- User threads execute in this mode.
Examples of privileged instructions: I/O instructions, halt, reset, mask
interrupts, set interval timer, set status register, and modify page table
registers.
Differences, similarities between interrupts, traps, system calls?
Schema of System operation:
- System powered on; in supervisor mode.
- System boots, kernel initializes, still in supervisor mode.
- System enters user mode to run user threads.
Should there be a user mode instruction to enter supervisor mode?
How can supervisor mode be re-entered?
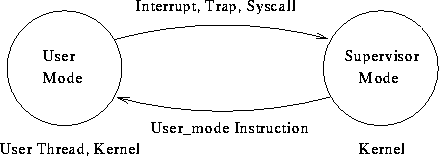
Prevent thread from scribbling on arbitrary memory locations.
Mechanisms:
- Bounds registers:
- Base, limit registers.
- Base, length registers.
How does it work?
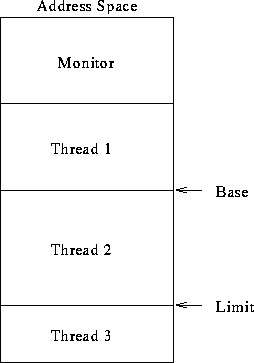
- Thread 2 running.
- All generated addresses checked against base, limit.
- ``Out-of-bounds'' addresses generate traps.
What needs to be done on a context switch?
- Virtual memory --- threads run in individual ``virtual'' address
spaces.
Virtual address space mapped onto subset of physical address space:
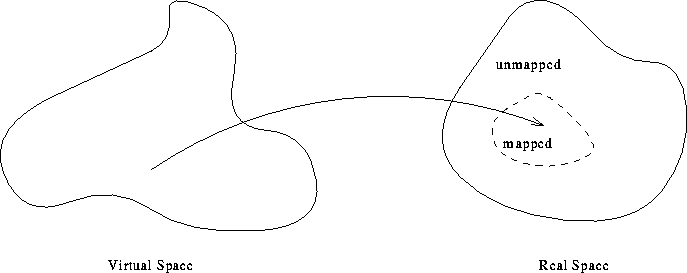
What prevents a thread from grabbing the CPU and not relinquishing it?
Interval timer, interrupt. On context switch:
- Set interval time.
- Run user thread.
- Timer interrupt generated on timer expiration.
- Run timer interrupt handler.
Used to:
- Enforce timesharing quantum. Typical quantum: 100 ms.
- Keep time (?!?).
How does a process perform I/O if it's a privileged operation?
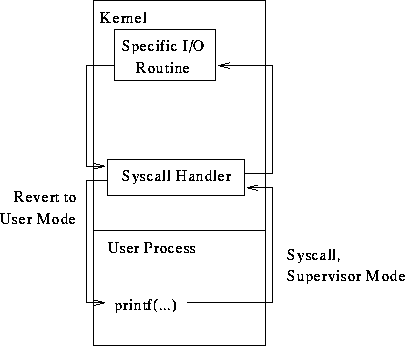
Thomas P. Kelliher
Wed Jan 21 14:02:08 EST 1998
Tom Kelliher