Process Resources; CPU Scheduling
Tom Kelliher, CS 318
Feb. 25, 1998
Announcements:
From last time:
- Lamport's bakery algorithm.
- ``Classic'' synchronization problems.
Outline:
- Process resources.
- Process scheduling.
- Context switching.
- Process operations.
Assignment: Read Chapter 5.
- Program in execution.
- Serial, ordered execution within a single process. (Contrast
task with multiple threads.)
- ``Parallel'' unordered execution between processes.
Three issues to address
- Specification and implementation of processes --- the issue of
concurrency (raises the issue of the primitive operations).
- Resolution of competition for resources: CPU, memory, I/O
devices, etc.
- Provision for communication between processes.
Three possible states for a process:
- Running --- currently being executed by the processor
- Ready --- waiting to get the processor
- Blocked (waiting) --- waiting for an event to occur: I/O
completion, signal, etc. (Suspended --- ready to run but not eligible.)
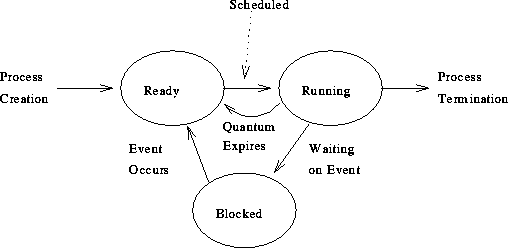
How many in each state?
Kept in a process control block (PCB) for each process:
- Code (possibly shared among processes).
- Execution stack --- stack frames.
- CPU state --- general purpose registers, PC, status register, etc.
- Heap --- dynamically allocated storage.
- State --- running, ready, blocked, zombie, etc.
- Scheduling information --- priority, total CPU time, wall time, last
burst, etc.
- Memory management --- page, segment tables.
- I/O status --- devices allocated, open files, pending I/O requests,
postponed resource requests (deadlock avoidance).
- Accounting --- owner, CPU time, disk usage, parent, child processes,
etc.
Contrast program.
PCB updated during context switches (kernel in control).
Should a process be able to manipulate its PCB?
Determination of which process to run next (CPU scheduling).
Multiple queues for holding processes:
- Ready queue --- priority order.
- I/O queues --- request order.
Consider a disk write:
- Syscall.
- Schedule the write.
- Modify PCB state, move to I/O queue.
- Call short term scheduler to perform context switch.
Is it necessary to wait on a disk write?
- Event queues --- waiting on child completion, sleeping on timer, waiting
for request ( inetd).
Three types of schedulers:
- Long term scheduler.
- Medium term scheduler.
- Short term (CPU) scheduler.
Determines overall job mix:
- Balance of I/O, CPU bound jobs.
- Attempts to maximize CPU utilization, throughput, or some other
measure.
- Runs infrequently.
Cleans up after poor long term scheduler decisions:
- Over-committed memory --- thrashing.
- Determines candidate processes for suspending and paging out.
- Decreases degree of multiprogramming.
- Runs only when needed.
Decides which process to run next:
- Picks among processes in ready queue.
- Priority function.
- Runs frequently --- must be efficient.
Time line schematic:
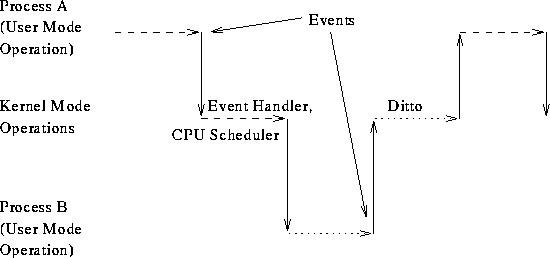
Parent, child.
Where does the child's resources come from? By ``resources'' we mean:
- Stack.
- Heap.
- Code.
- Environment --- environment variables, open files, devices, etc.
Design questions:
- New text, same text?
- Make a copy of the memory areas? (Expensive.)
- Copy the environment?
- How are open files handled?
Solutions to the ``copy the parent's address space'' problem:
- Copy on write --- Mark all parent's pages read only and shared by
parent & child. On any attempted write to such a page, make a copy and
assign it to child. Fix page tables.
- vfork --- No copying at all. It is assumed that child will
perform an exec, which provides a private address space.
Thomas P. Kelliher
Mon Feb 23 08:03:04 EST 1998
Tom Kelliher