Compilation Example, Summary of Addressing Modes, and Other
Architectures
Tom Kelliher, CS 240
Feb. 3, 1999
Read Sections 4.1--4.3.
Branch instructions, control structures.
- Compilation example.
- Summary of addressing modes.
- PowerPC and 80x86.
Number representation, addition and subtraction.
I assume everyone can convert this to the equivalent while loop and
compile it. If not, see me.
for (i = 1; i <= 32 ; i *= 2)
cout << i << endl;
(Details: print int --- 1 in $v0
, value in $a0
; print string
--- 4 in $v0
, pointer to string in $a0
.)
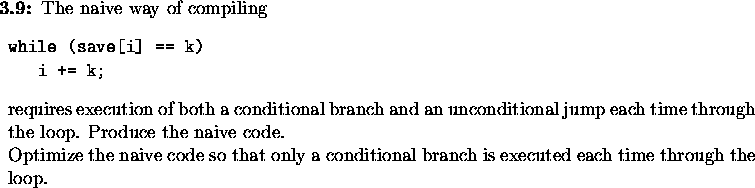
Effective address: location of the operand.
Possible operand locations:
- Immediate mode: operand is in the instruction.
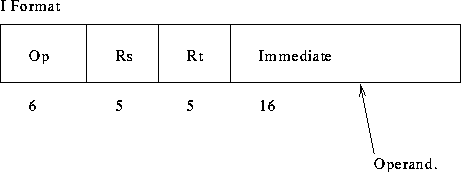
- Register mode: operand is stored in a register whose ID is in the
instruction.
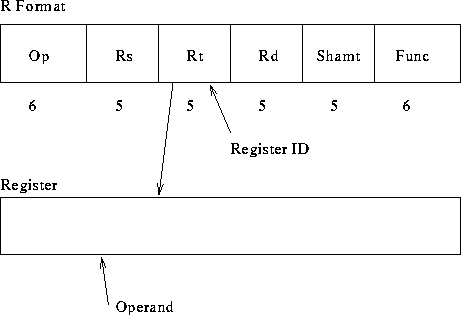
- Base/Offset: the memory address of the operand is the sum of a base
address (stored in a register whose ID is in the instruction) and a signed
immediate offset (stored in the instruction)
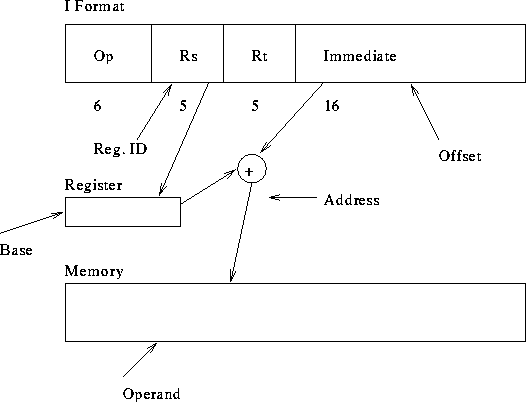
Synthesizing other addressing modes from this.
-
PC-relative: address is the sum of the PC and a signed immediate constant.
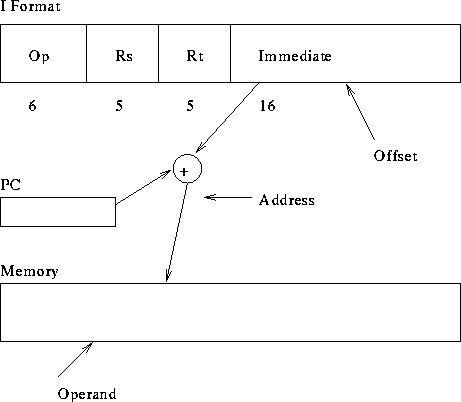
- Pseudo-Direct mode: address is the concatenation of the 4 msbs of the
PC, the address field, and 00.
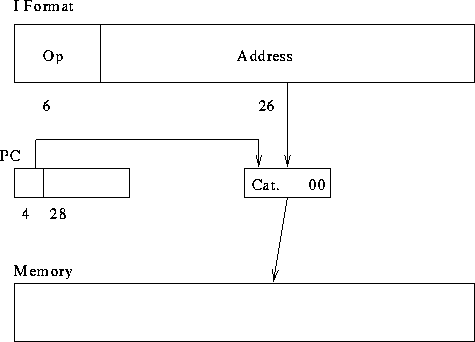
Basics similar to MIPS:
- 32 registers.
- 32-bit instructions.
- Load-Store architecture.
Additional features:
- Indexed addressing: address is sum of two registers.
General applicability?
- Update addressing: similar to base/offset addressing with the
additional wrinkle of automatically incrementing the base register by
sizeof(operandType) after access.
Other strides? ALU conflicts?
- Load/store multiple: load/store upto 32 registers in a single
instruction. Useful for: register save/restore, block memory copying.
Complexity? Can it be done with hardwired control?
- A special loop control register, distinct from the 32 registers and
accessed by a special conditional branch instruction which decrements it
and then tests it ( =0, !=0).
Useful for:
for (i = n; i != 0; --i)
/* code block */;
Not generally useful. What it can lead to.
MIPS was the vision of a single small group in 1985; the pieces of this
architecture fit nicely together, and the whole architecture can be
described succinctly. Such is not the case for the 80x86; it is the
product of several independent groups who evolved the architecture over
almost 20 years, adding new features to the instruction set as someone
might add clothing to a packed bag.
Background:
- Technology in the late '70s.
- Players in the computing industry.
- 1981: the IBM PC and Intel's changing fortunes.
Motorola and the 68K.
Implementation history:
- 8086 as a 16-bit extension of the 8080.
The ``hamstrung'' 8085.
Support for IEEE floating point.
- 80186: The forgotten CPU.
- 80286: 24-bit addressing, MMU, protected mode.
The protected mode kludge.
- 80386: 32-bit processor, real protection.
Finally, we can run Unix on it.
- 80486, Pentium, and Pro: Virtually no architectural changes.
- MMX: SIMD-style small data instructions.
Basics:
- 32-bit data and address.
- Two-address architecture.
- Operand combinations: register/register, register/immediate,
register/memory, memory/register, memory/immediate.
- 8 32-bit ``general purpose'' registers.
- Several 16-bit registers: code and stack segment pointers, several
data segment pointer.
- Condition codes register. Conditions codes set as a side-effect of
ALU instructions.
Advantage: moves the compare from the branch to the ALU instruction.
Disadvantage: superscalar, OOE.
- Variably-sized instructions: 1 to 17 bytes. Example prefix bytes:
- Override default segment register.
- Lock the memory bus.
- repeat instruction until ECX clears.
Addressing modes:
- Some instructions use postbytes to specify addressing modes.
New addressing modes:
- Register indirect.
Direct MIPS synthesis.
- Base with 8- or 32-bit offset.
- Base with scaled index
- Base with scaled index and 8- or 32-bit offset.
Thomas P. Kelliher
Tue Feb 2 13:35:04 EST 1999
Tom Kelliher