ADT Binary Tree
Tom Kelliher, CS23
May 7, 1997
A tree:
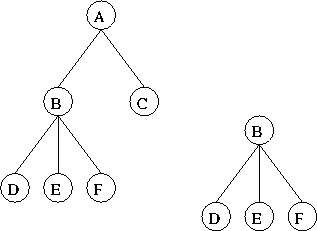
- Vertex/node , directed edge.
- Root, interior vertex, leaf.
- Parent, child; ancestor, descendant.
- Siblings.
- Subtrees.
- General trees.
- A Binary tree, T, is a set of nodes such that either:
- T is empty.
- T is partitioned into three sets:
- A single root.
- Two possibly empty sets that are binary trees, the left and
right subtrees of the root.
- Binary search tree:
- Each vertex has a label.
Consider a node labeled r:
- If q is in r's left subtree, q is less
than r.
- If q is in r's right subtree, q is
greater than r.
- Height, level.
- Full, complete. The book's defn. of complete is wrong.
Counterexample?
- Balanced binary tree, completely balanced binary tree.
Recursive structure.
- CreateBinaryTree()
- CreateBinaryTree(RootItem)
- CreateBinaryTree(RootItem, LeftTree, RightTree)
- DestroyBinaryTree()
- RootData()
- SetRootData(NewItem)
- AttachLeft(NewItem, Success)
- AttachRight(NewItem, Success)
- AttachLeftSubtree(LeftTree, Success)
- AttachRightSubtree(RightTree, Success)
- DetachLeftSubtree(LeftTree, Success)
- DetachRightSubtree(RightTree, Success)
- LeftSubtree()
- RightSubtree()
- PreorderTraverse(Visit)
- InorderTraverse(Visit)
- PostorderTraverse(Visit)
typedef char NodeType;
struct NodeItem
{
NodeItem* left, right;
NodeType item;
};
typedef NodeItem* Node;
void Preorder(Node T, void (*Visit)(NodeType))
{
if (T != NULL)
{
Visit(T->item);
Preorder(T->left, Visit);
Preorder(T->right, Visit);
}
}
void Preorder(Node T, void (*Visit)(NodeType))
{
if (T != NULL)
{
Inorder(T->left, Visit);
Visit(T->item);
Inorder(T->right, Visit);
}
}
void Preorder(Node T, void (*Visit)(NodeType))
{
if (T != NULL)
{
Postorder(T->left, Visit);
Postorder(T->right, Visit);
Visit(T->item);
}
}
void visit(NodeType n)
{
cout << n << endl;
}
Assume each function is called on the root of:
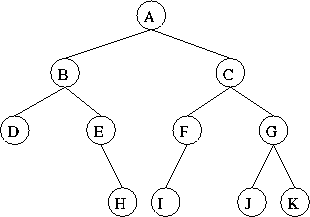
What is printed?
Been there, done that.
How do we get to the parent from the child?
- Tree must be complete.
- Must know number of vertices.
The tree:
char tree[MAXTREE]; // tree[0] is root
children of tree[i]
:
- Left child:
tree[2 * i + 1]
- Right child:
tree[2 * i + 2]
Can get to parent from child.
Thomas P. Kelliher
Tue May 6 15:54:24 EDT 1997
Tom Kelliher