Computer Execution, SAL
Tom Kelliher, CS 220
Sept. 15, 1997
Read 2.4--2.6.
How is a program executed?
How does it look?
Where does it reside?
Similar to machine language.
consider HLL code:
a = 2 * b + c;
In assembly language, this might appear:
mult temp, b, 2
add a, temp, c
What do we see here:
- HLL, assembly statement correspondence.
- Opcode corresponding to machine instructions.
- Labels referring to memory locations.
Another example. HLL:
if (a == b)
c = d;
else
c = e;
Assembly:
if: bne a, b, else
add c, d, 0
b endif
else: add c, e, 0
endif:
Classes of instructions:
- Arithmetic, logic.
- Control: conditional branch, unconditional branch, procedure
call/return, interrupt, machine control.
- Data transfer: memory load/store, I/O.
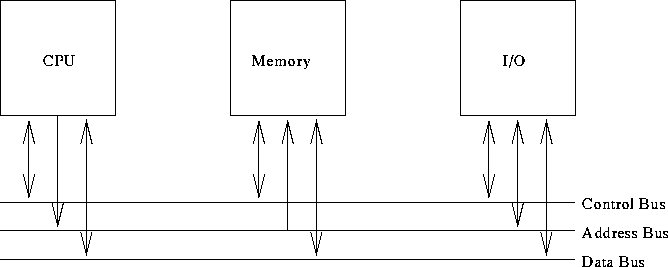
Machine/assembly instructions are distinct and are executed
``individually.''
Program counter (PC): a register holding the address of the next
instruction to be executed.
The cycle:
- Fetch (read) the instruction pointed to by the PC.
- Decode the instruction and increment the PC. (By how much?)
- Fetch operands.
- Execute.
- Store (write) results.
How is this modified for a conditional branch?
Modifications for modern CPUs:
- Pipelining.
- Multiple-Issue.
- Out of order execution.
With what must a programming language provide us?
- Primitive data types.
- Sequential execution. Implicit in most instructions.
- Conditional execution. Conditional branches.
- Iterative execution. Conditional and unconditional branches.
- I/O. I/O instructions.
Assembler directives.
Declared in a .data segment. Example:
.data
foo: .word 345
bar: .byte 'c'
pi: .float 3.14159
- Label, value optional.
- Why would label be optional?
- .word
- .byte
- .float
Instructions go into a .text segment. Execution begins with the
instruction labeled __start
.
Example:
add a, b, c
- Opcode.
- Destination, source operands.
- 3-address architecture.
First set of AL instructions:
- move --- 2 address.
- add, sub, mul, div, rem --- 3 address.
- Integer division, remainder. Arithmetic rules.
Thomas P. Kelliher
Sun Sep 14 17:26:01 EDT 1997
Tom Kelliher