Instruction Set Design
Tom Kelliher, CS 220
Nov. 14, 1997
Questions on the homework assignment?
Handling of the final assignment (postfix calculator): completion deadline,
optional milestone deadlines.
We descend deeper.
To execute, a program's instructions must be in memory.
What do instructions look like?
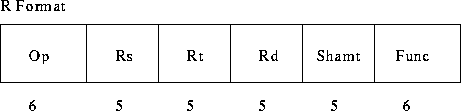
Design choices for the architect:
- Fixed-, variable length instructions.
- Small, large instructions.
- Addressing modes.
- Number and type of instructions.
- Number of operands.
- Size of register file. (32 for MIPS.)
Tradeoffs:
- Silicon real estate.
- Simplified decoding.
- Reduced fetch bandwidth.
- Instruction complexity.
- Life before and after caches.
Abbreviated RISC design philosophy:
- Instructions should be kept simple unless there is very good reason to
do otherwise.
- Simple decoding and pipelined execution are more important than
program size.
RISC CPU traits:
- Load/store; operations are register-register. Helps to reduce
instruction size. Memory-memory operations are not that common given a
large register file. Consider:
.data
a: .word
b: .word
c: .word
.text
add a, b, c
If addresses are 32-bits, how large is the add instruction?
- The operations and addressing modes are reduced.
- Instruction formats are simple and do not cross word boundaries.
- RISC branches avoid pipeline penalties.
Programmer-visible hierarchy:
- Registers.
- Main memory.
- Disk.
- ...
Some parts of the hierarchy are not programmer visible.
Consider the C code:
a = b + c;
Three address:
add a, b, c # SAL
lw $8, b($0) # MAL Inefficient.
lw $9, c($0)
add $8, $8, $9
sw $8, a($0)
Two address:
move a, b
add a, c
One address (implied operand --- accumulator):
load a
add b
store c
Zero address (stack machine):
push a
push b
add
pop c
What are the advantages/disadvantages? Consider the C code:
ave = (a + b + c + d) / 4;
How many instructions are required for each number of operands?
Sizes of instructions?
Effective address: location of the operand.
Possible operand locations:
- Immediate mode: operand is in the instruction.
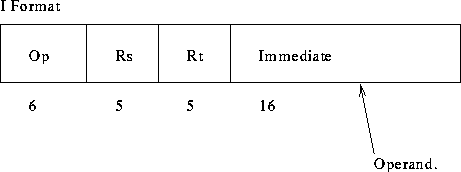
This mode is used for constants.
- Direct mode: the memory address of the operand is in the instruction.
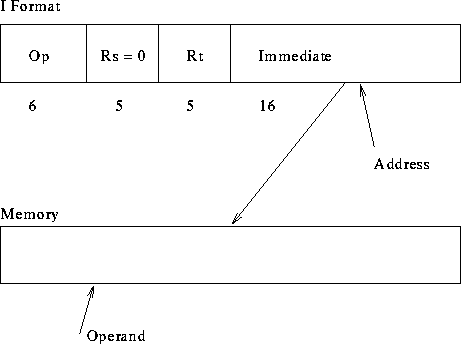
This is the model we associate with SAL.
- Register mode: operand is stored in a register whose ID is in the
instruction.
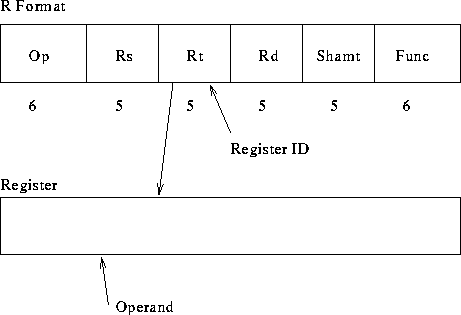
Similar to direct mode, but faster and a far smaller ``memory.''
- Register direct mode: the memory address of the operand is stored in
a register whose ID is in the instruction.
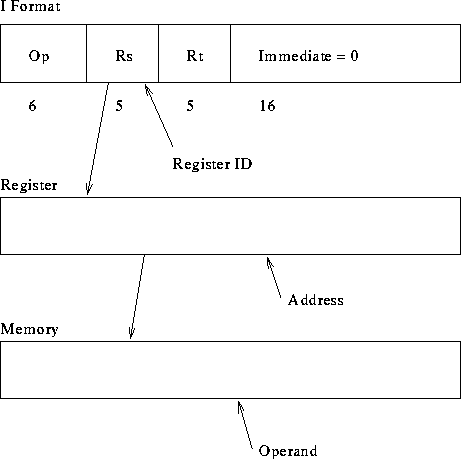
The register is a pointer.
- Base Displacement: the memory address of the operand is the sum of a
base address (stored in a register whose ID is in the instruction) and an
immediate displacement (stored in the instruction)
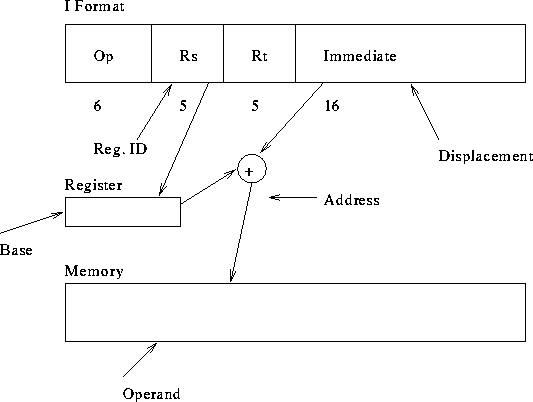
Displacements are signed.
Array, struct access. Not so much array.
Branch instructions use PC-relative addressing, which is similar to Base
displacement:
- PC is base.
- Displacement is stored as a signed immediate in the instruction.
- When branch taken, computed address is stored into PC.
The MIPS combines three of these into one! Can you tell which three?
Thomas P. Kelliher
Thu Nov 13 16:55:38 EST 1997
Tom Kelliher