Homework 2
CS17
40 pts.
- Design pseudocode to control a soda machine. (As an aside, why
wouldn't we refer to this pseudocode as an algorithm?) The machine
stocks 4 kinds of soda: Coke (50 cents), Diet Coke (50 cents), root beer
(65 cents --- it's really good stuff), and Mountain Dew (40 cents,
the favorite of hackers).
Inputs:
- Coins: nickels, dimes, quarters
- Button pushes, corresponding to the 4 kinds of soda
- Indications from the four soda compartments as to whether or not each
one is empty
Outputs:
- A soda (possibly)
- Change (possibly)
I want you to do the requirements specification, analysis, and design steps
for this problem. Your design is the most important part. Your pseudocode
design should demonstrate top-down stepwise refinement.
Wait in a continuous loop, monitoring the machine:
- Coin drops
- Soda available
- Button pushes
The machine dispenses soda and returns any required change.
Inputs:
- Coins: nickels, dimes, quarters
- Button pushes, corresponding to the 4 kinds of soda
- Indications from the four soda compartments as to whether or not each
one is empty
Outputs:
- A soda (possibly)
- Change (possibly)
Conditions:
- Coke and Diet Coke are 50 cents, Mountain Dew is 40 cents, and root
beer is 65 cents.
- If change equal to at least the amount of the soda desired has been
entered, a soda will be dropped (if one is available), and any change
returned.
- If a soda is not available, or insufficient change has been put into
the machine, the change is returned.
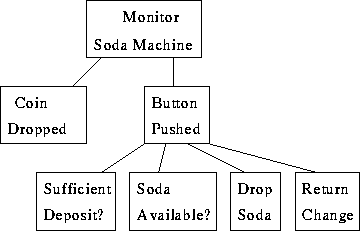
let deposit = 0
while forever
if quarter dropped
let deposit = deposit + 25
else if dime dropped
let deposit = deposit + 10
else if nickel dropped
let deposit = deposit + 5
else if mountain dew selected
if deposit >= 40 and mountain dew is available
drop mountain dew
let deposit = deposit - 40
end_if
return remaining deposit
let deposit = 0
else if coke selected
if deposit >= 50 and coke is available
drop coke
let deposit = deposit - 50
end_if
return remaining deposit
let deposit = 0
else if diet coke selected
if deposit >= 50 and diet coke is available
drop diet coke
let deposit = deposit - 50
end_if
return remaining deposit
let deposit = 0
else if root beer selected
if deposit >= 65 and root beer is available
drop root beer
let deposit = deposit - 65
end_if
return remaining deposit
let deposit = 0
end_if
end_while
Thomas P. Kelliher
Mon Mar 4 08:08:51 EST 1996
Tom Kelliher