Computers, Program Execution, and Data Representation
Tom Kelliher, CS 102
Jan. 25, 1999
First quiz on Friday.
Introductory exercises.
Survey
- Program execution.
- A model computer.
- Data representation.
MS Word --- bring the workbook.
Consider the following simple program:
1: let sum = 0
2: print "How many numbers? "
3: read count
4: let loopCount = count
5: if loopCount equals 0 goto 11
6: print "Next number: "
7: read input
8: let sum = sum + input
9: let loopCount = loopCount - 1
10: goto 5
11: let average = sum / count
12: print "The average is:", average, "."
13: end
- Where do we begin?
- After completing one step, where do we proceed?
- Operations: assignment, arithmetic, decision, branch, I/O.
- Operands: Variables, constants (numeric and string).
A block diagram:
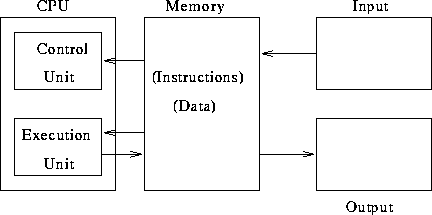
- What is memory? How does it differ from storage?
- What parts of a PC are considered input devices? Output devices?
Both?
- Computers use the binary system. Why?
- Binary digits.
- Bits, bytes, words.
- Memory locations: cells with addresses.
- Converting between binary and decimal:
- Binary to decimal: Consider some examples. 1, 10, 100, 110. What
to do in general?
- Decimal to binary: We need a little algorithm.
read decimalNumber
while decimalNumber does not equal 0
{
let remainder be the remainder of decimalNumber / 2
let decimalNumber be the integer quotient of decimalNumber / 2
print remainder to the left of any other digits printed
}
- How do we represent characters? ASCII code:
- A: 01000001
- 4: 00110100
- How does the computer know if a memory location contains numbers,
characters, variables, or instructions?
Thomas P. Kelliher
Sun Jan 24 18:45:59 EST 1999
Tom Kelliher