PHP: Sessions and PostgreSQL Connectivity
Tom Kelliher, CS 318
Feb. 18, 2002
Catch up on the reading!!!
SQL queries.
- Introduction.
- Sessions.
- PostgreSQL connectivity.
- Example code walk-through.
PHP/PostgreSQL lab.
- HTTP is a stateless protocol.
- What does this mean?
- What are the consequences?
- Mechanisms for retaining state (persistence):
- Hidden fields in forms.
- Cookies.
- Sessions.
Advantages, disadvantages.
- HTTP/PHP session information transfer model:
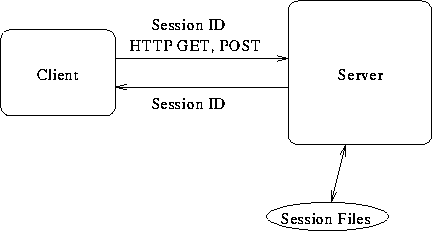
- HTTP GET: parameters passed as part of URL:
http://phoenix.goucher.edu/process.php?name=tom
- Accessed through
_GET
associative array in PHP:
$name = $_GET["name"];
- Session ID passed as GET parameter:
echo "<A href=\"http://phoenix.goucher.edu/process.php?"
. SID . "\">";
- HTTP POST: parameters passed into script via
stdin
.
- Accessed through
_POST
associative array.
- Session variables are maintained on the server and accessed by
referring to a session ID and using the
_SESSION
associative
array.
- Sessions exist until browser is closed or PHP garbage collector
removes the session data file.
- Establishing a session and writing session variables:
session_start();
$_SESSION["username"] = $username;
$_SESSION["password"] = $password;
-
session_start()
and new/resumed sessions.
- The session ID constant:
SID
.
- Checking to see if a session variable already exists:
if (isset($_SESSION["username"])
$username = $_SESSION["username"];
else
$_SESSION["username"] = $username;
- Deleting a session variable (enhanced security):
unset($_SESSION["username"]);
Also possible to delete entire session --- see online docs.
- Avoiding garbage collection:
- Garbage collector invoked by any
session_start()
.
- Session files older (mod time) than 24 minutes are reclaimed.
- Avoiding garbage collection? Read/write a session variable.
- Processing model:
- Establish connection, receive handle.
- Send SQL query, receive results ``array.''
- Process results array.
- Free results array.
- Repeat as needed.
- Close connection.
- Establishing a connection:
$handle = pg_connect("dbname=databaseName user=userName password=pwd");
Check handle status!! Why handles? (Script could have multiple DB
connections open.)
- Sending a query:
$result = pg_exec($handle, "query string");
Check result status!!
- Determining the size of a result:
pg_numrows($result)
,
pg_numfields($result)
.
- Accessing the result:
$item = pg_result($result, $row, $field);
$item = pg_result($result, $row, "fieldName");
$row
and $field
are 0-based numeric indices. fieldName
is an associative array-style index.
- Freeing a result, closing a connection:
pg_freeresult($result);
pg_close($handle);
Refer to Class Materials section of course web site.
Things to note for each file:
-
login.html
:
- Form tag: method and action.
- Input tags: types and names.
-
authenticate.php
:
- Debugging notes.
- Retrieval of username, password. Associative array.
- Database connection and error checking.
- Sending a query and error checking.
- Accessing query results. Associative array.
Why the check on pg_numrows()
?
- Establishing the session and saving session variables.
- Passing
SID
back to the server as a GET parameter.
SID
will be empty when we resume the session.
- Freeing the result and closing the database. Why?
-
query.php
:
- Retrieving session variables.
- Iterating through the result.
Thomas P. Kelliher
Thu Feb 14 18:09:51 EST 2002
Tom Kelliher