Overview of Java, JWS
Tom Kelliher, CS 116
Sept. 4, 2000
Workload discussion (Section 1).
Handout: the twelve recurring concepts.
Study Section 1.5 and the lab handout.
Introduction, background.
- Overview of Java.
- Java Workshop.
Lab 1.
- Applications: standalone programs.
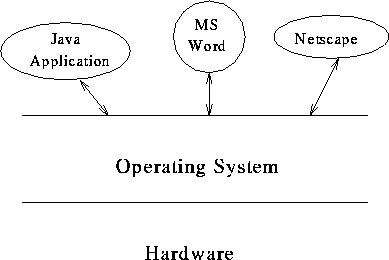
- Applets: operate within a web browser.
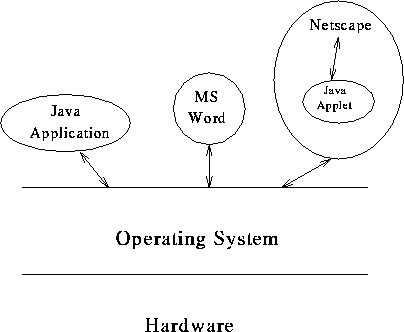
Recurring concept: Levels of Abstraction.
- HTML:
<HTML>
<HEAD>
<TITLE>
Hello World Java Applet
</TITLE>
</HEAD>
<BODY>
Here's my applet...
<HR>
<APPLET code="Hello.class"
width=150
height=60>
</APPLET>
<HR>
</BODY>
</HTML>
- Java applet:
import java.applet.*;
import java.awt.*;
// The Hello class simply displays the string "Hello, world!"
// within the graphics object.
public class Hello extends Applet
{
public void paint(Graphics g)
{
g.drawString("Hello, world!", 20, 10);
}
}
Run
the applet.
- Modular programming.
Basic, Pascal, C.
Control flow model. Flowcharting, pseudo-code.
- Object-Oriented programming (OOP)
- Definition: A programming methodology that is centered upon the
notion of objects which communicate action requests back and forth.
- Object: A collection of private data and methods (actions)
manipulating the object (actually, its data).
- Class: All objects belong to a class. Our Java programs declare
and define the classes. Once we define a class, we can begin to use it
by declaring objects to be of that class.
So, objects also have (unique) names.
- Inheritance allows us to reuse data and methods from one
class in another class. This is
extends
, although we're really
limiting the scope of the class. Also: binding, because we're
adding detail.
- Libraries: Classes that Java provides for us. We incorporate them
with the
import
statement. What concept(s)?
- An example. Wheww, finally. Work on this in groups of two or three:
Suppose we want to model cars: Tauruses, Civics, etc.
- Private data?
- Methods (operations)?
What class do these objects fit into?
Can we find a hierarchy of classes? (Inheritance) (Start with
vehicle.)
Demonstration:
- Creating a new project; adding an existing file.
- Compiling the project.
- Running the project. Bytecode interpreter.
Thomas P. Kelliher
Fri Sep 1 18:16:31 EDT 2000
Tom Kelliher